How to join a user to a discord server, get a list of their servers (PHP, OAuth2.0)
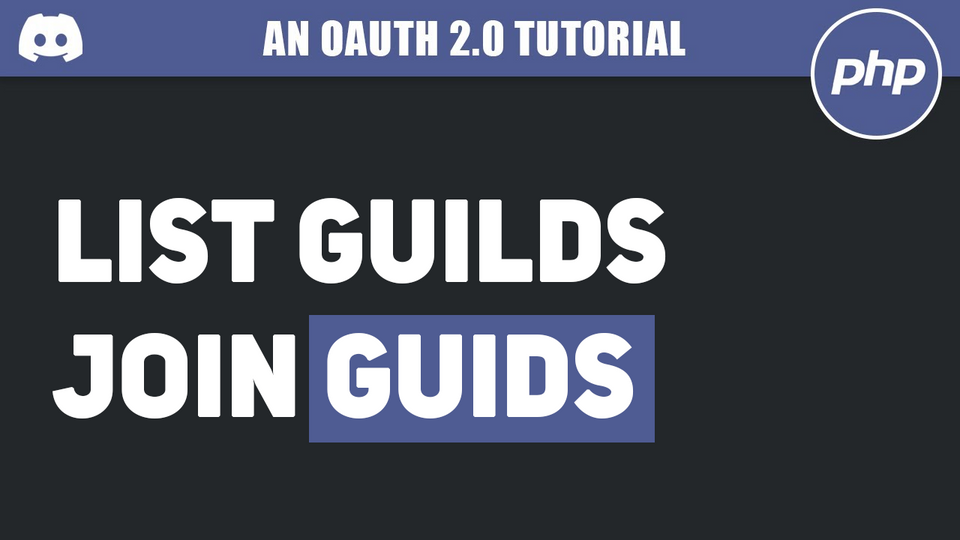
I've been getting a lot of requests to get a list of a user's guilds on discord, and all you have to do is modify the original login with discord code a little bit.
Video
Written Guide
Here are a list of changes
- Add scopes to the URL
- Add a joinGuild function
- Add a getGuildsList function
- Create a bot, add it to your server, and give it the right permissions
- Markup the user's guilds
So, if you haven't' already, we will start at the last tutorial's end:
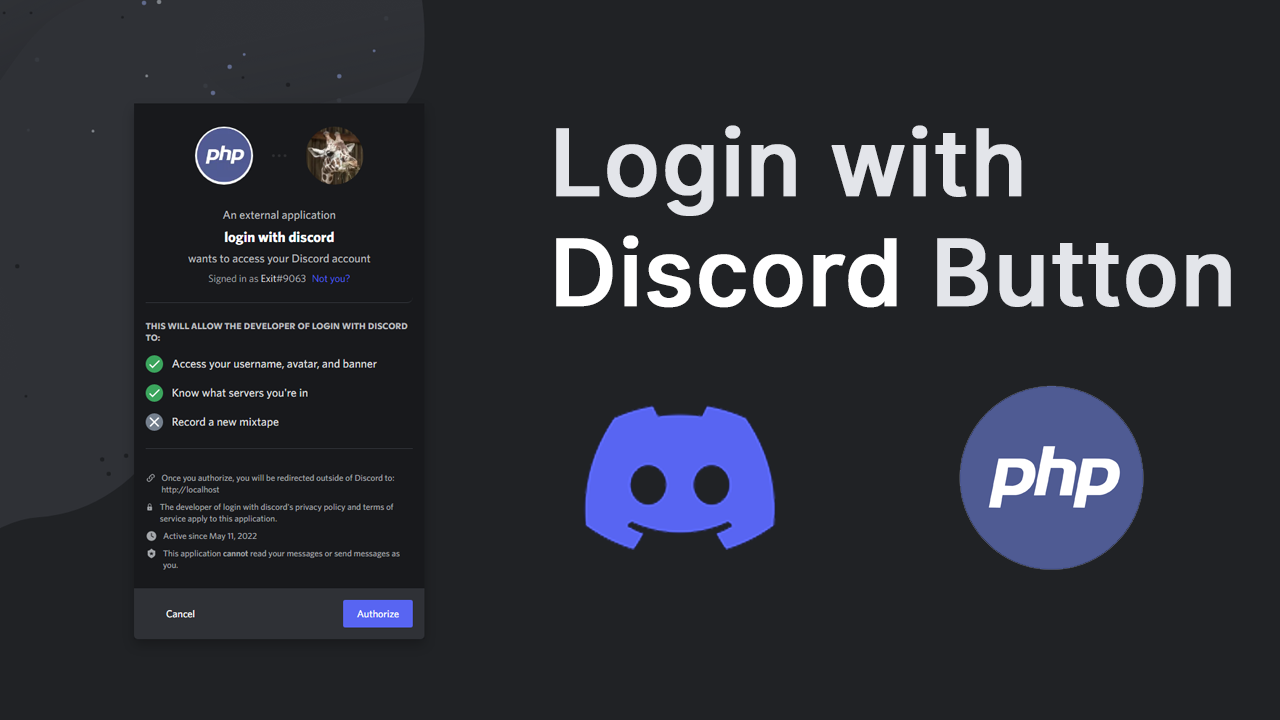
Getting a new URL
Generate yourself a new URL:
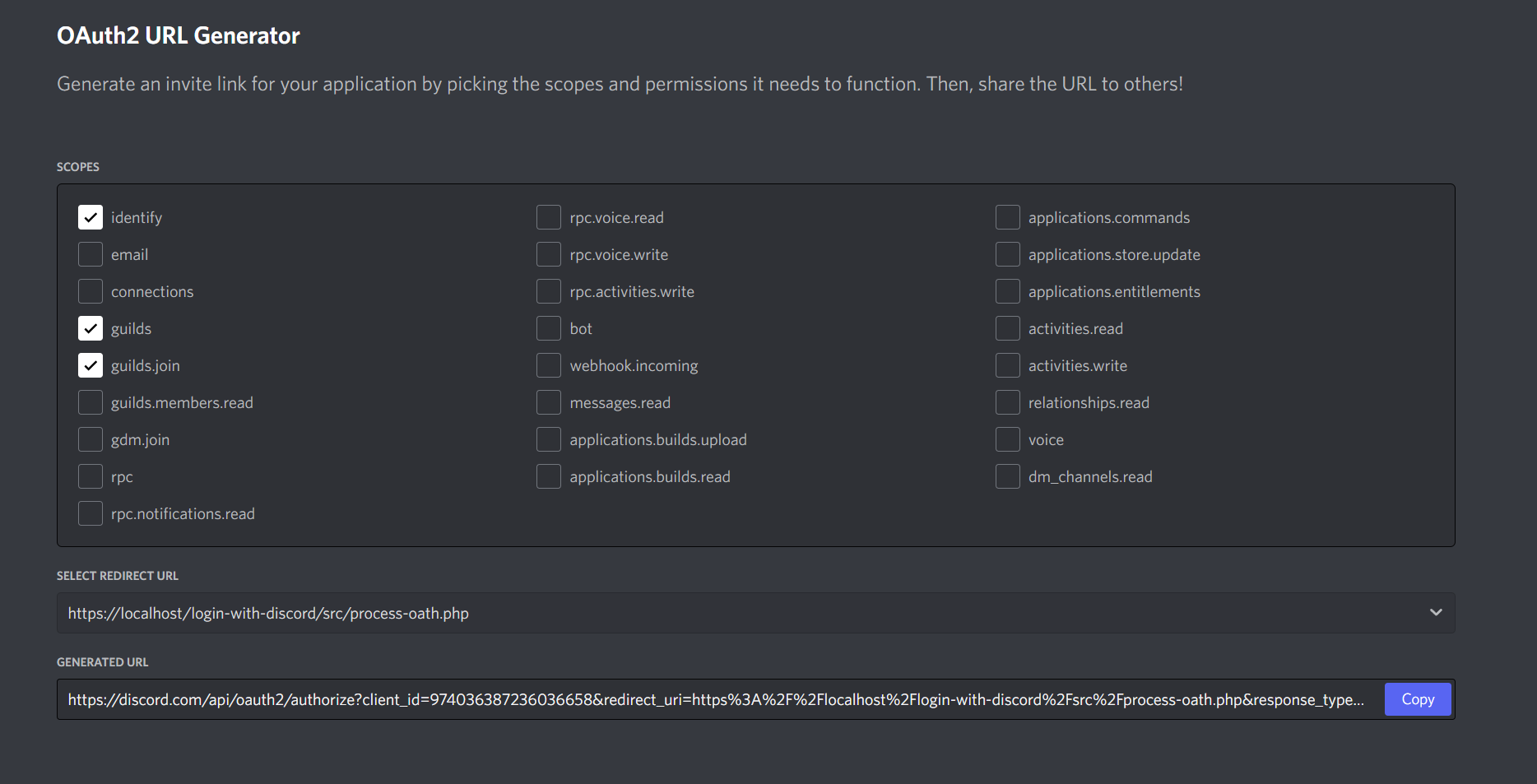
Update your init-oauth.php file
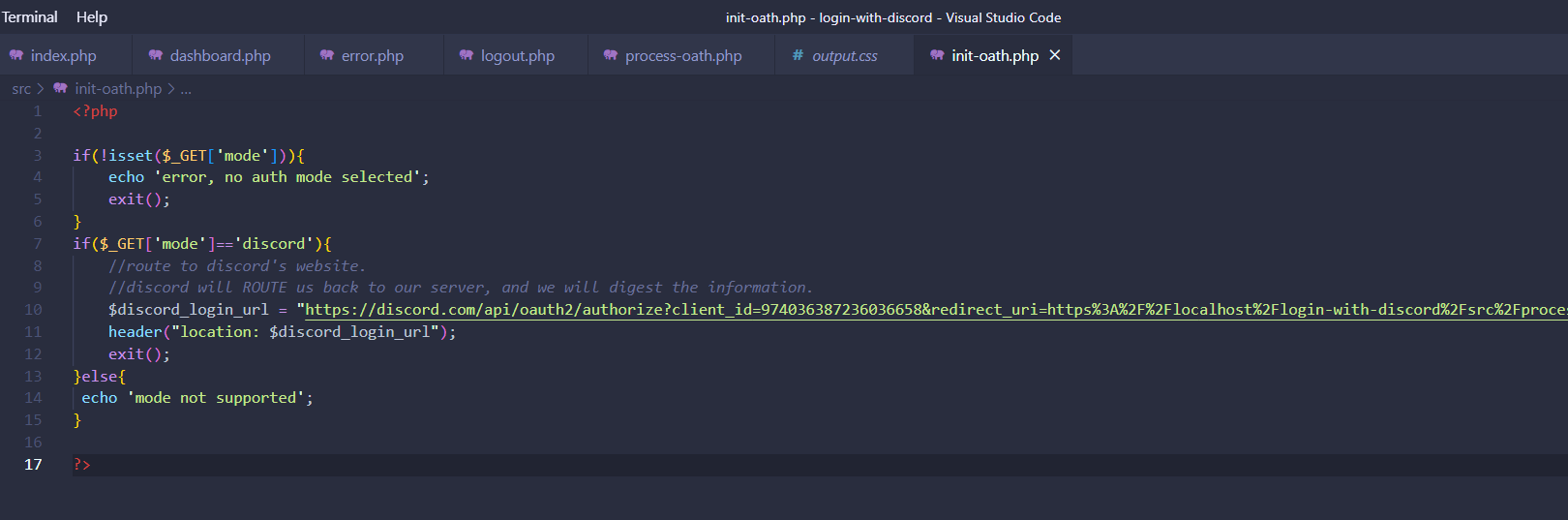
Handling the bot
Create the bot:
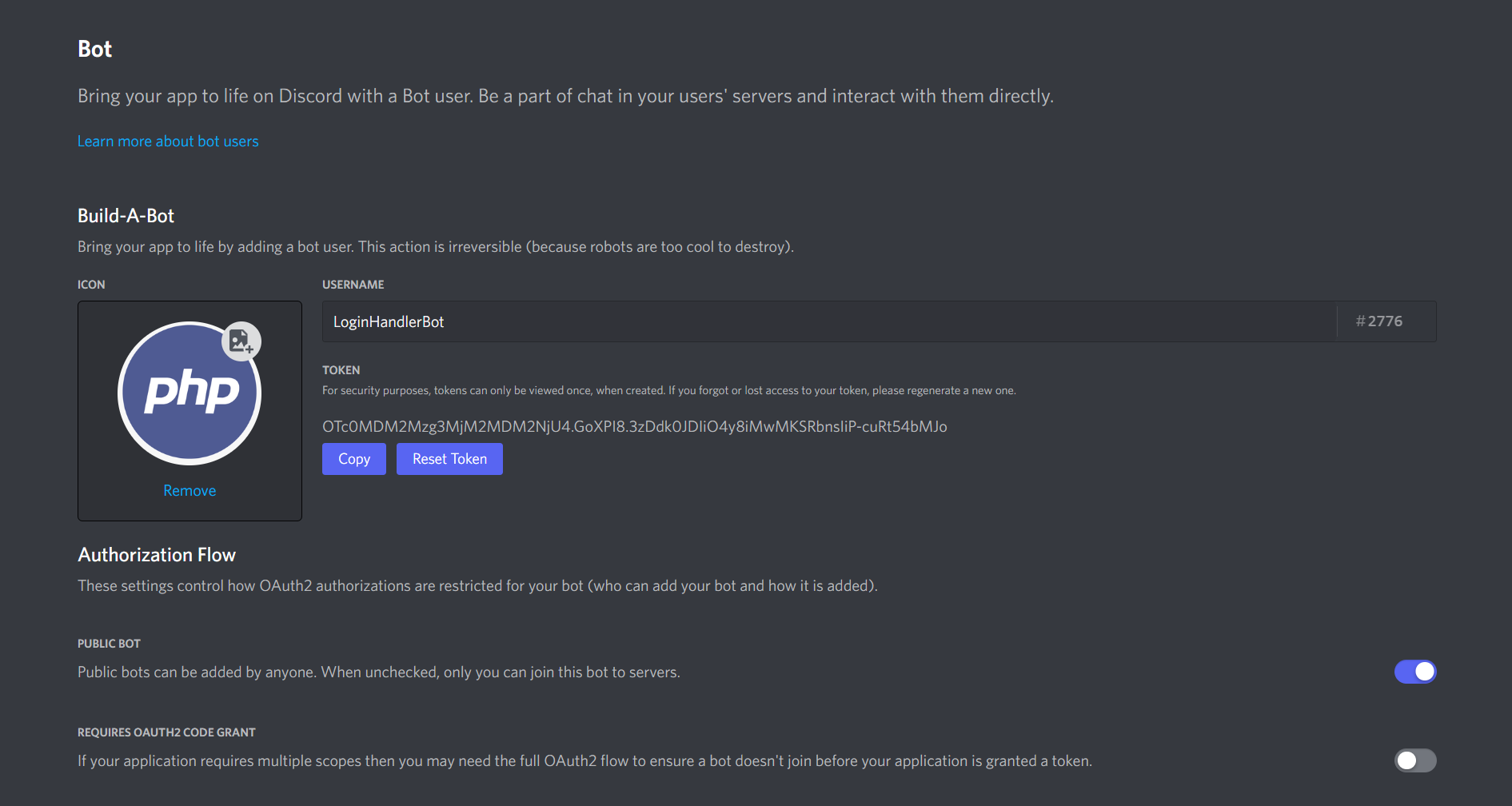
Create the bot by heading to the https://discord.com/developers/applications/ and going to the same application that handles your OAuth. Create the bot.
Invite the bot to your server:
Go to OAuth, URL Generator, and select all of these scopes (this will give your bot permission to read the oauth data, and add the user via bot)
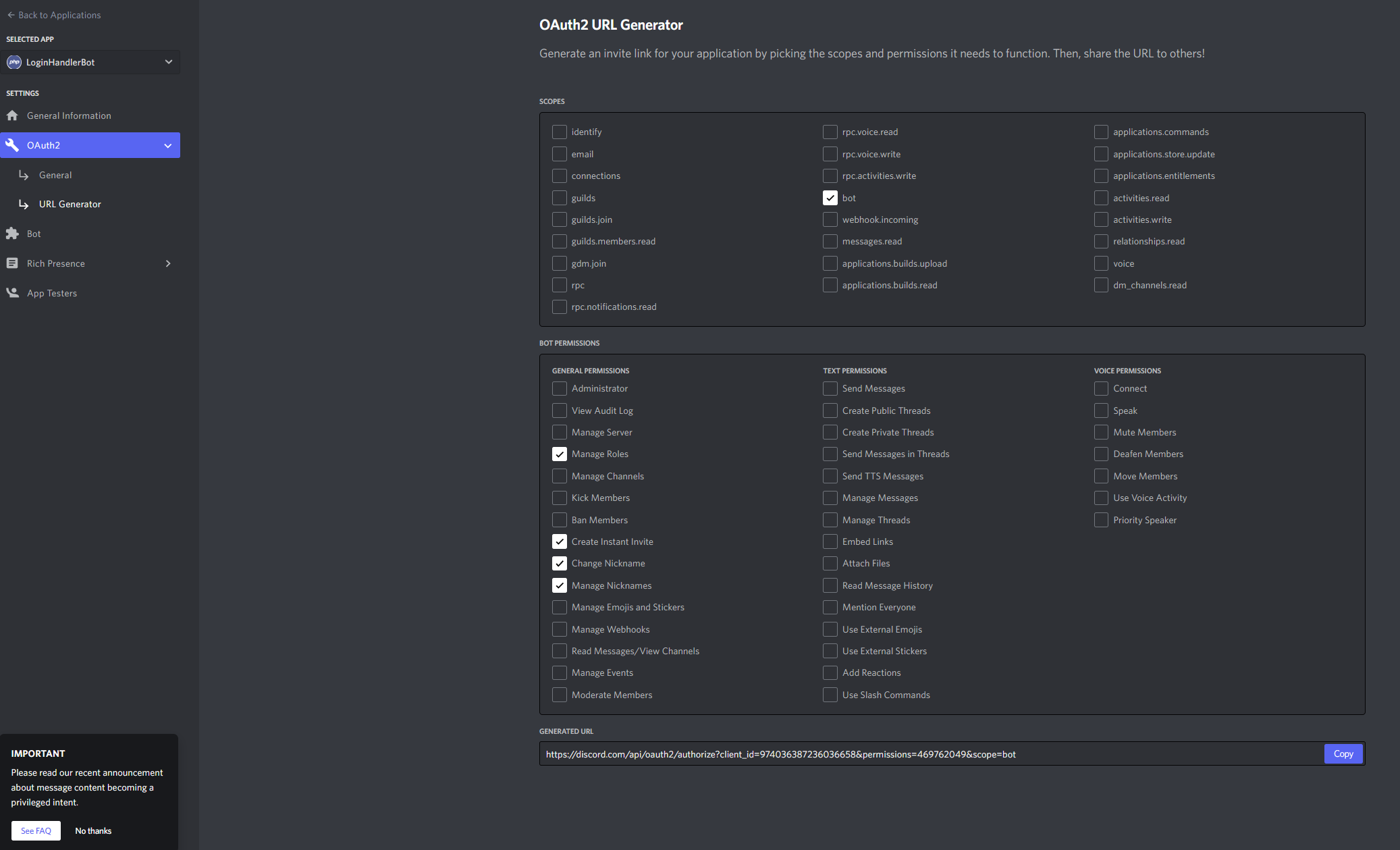
Finally, click on the link as the server owner, and add the bot to your server!
Adding Process Functions
Then, head on over to the proccess-oauth.php file and add the two new functions:
function addUserToGuild($discord_ID,$token,$guild_ID){
$payload = [
'access_token'=>$token,
];
$discord_api_url = 'https://discordapp.com/api/guilds/'.$guild_ID.'/members/'.$discord_ID;
$bot_token = "YOUR BOT TOKEN (SAME AS APPLICATION)";
$header = array("Authorization: Bot $bot_token", "Content-Type: application/json");
$ch = curl_init();
//set the url, number of POST vars, POST data
curl_setopt($ch, CURLOPT_HTTPHEADER,$header);
curl_setopt($ch,CURLOPT_URL, $discord_api_url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "PUT"); //must be put for this method..
curl_setopt($ch,CURLOPT_POSTFIELDS, json_encode($payload)); //must be a json body
curl_setopt($ch,CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0);
$result = curl_exec($ch);
if(!$result){
echo curl_error($ch);
}else{
return true;
}
}
function getUsersGuilds($auth_token){
//url scheme /users/@me/guilds
$discord_api_url = "https://discordapp.com/api";
$header = array("Authorization: Bearer $auth_token","Content-Type: application/x-www-form-urlencoded");
$ch = curl_init();
//set the url, number of POST vars, POST data
curl_setopt($ch, CURLOPT_HTTPHEADER,$header);
curl_setopt($ch,CURLOPT_URL, $discord_api_url.'/users/@me/guilds');
curl_setopt($ch,CURLOPT_POST, false);
//curl_setopt($ch,CURLOPT_POSTFIELDS, $fields_string);
curl_setopt($ch,CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0);
$result = curl_exec($ch);
$result = json_decode($result,true);
return $result;
}
The first function is a little bit tricky. We had to change the method to PUT using the CURLOPT_CUSTOMREQUEST parameter. Additionally, it insisted it wanted a json body so we encoded the token into that data format.
The getUsersGuilds is very similar to getting the user's data, however, this time it's /users/@me/guilds.
Now, let's actually use these functions in our code:
I'm adding the user to my guild when I also fetch their user data, here is the area:
$guild_ID = '986726427950989372';
$addUserToGuild = addUserToGuild($result['id'],$access_token,$guild_ID);
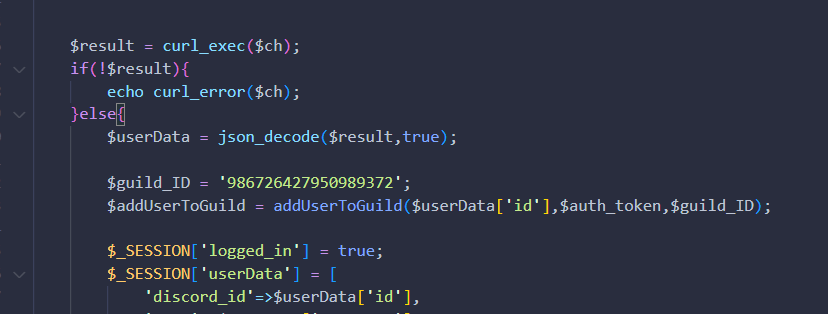
Additionally, if you want to fetch their guild data, I will just append the function into my session variable:
$_SESSION['userData'] = [
'discord_id'=>$userData['id'],
'name'=>$userData['username'],
'avatar'=>$userData['avatar'],
'guilds'=>getUsersGuilds($access_token)
];
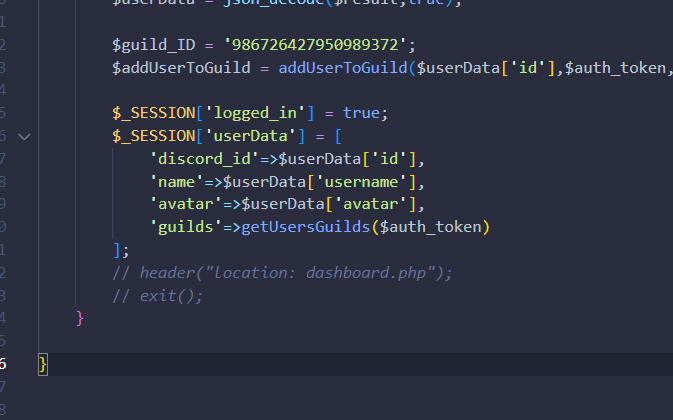
Finally, markup your new guild data:
Add this to the head of the file;
$guilds = $_SESSION['userData']['guilds'];
$guildMarkup='';
foreach ($guilds as $key => $guildData) {
$guildMarkup.='<li class="py-2 px-4 w-full rounded-t-lg border-b border-gray-200 dark:border-gray-600">'.$guildData['name'].'</li>';
}
And this to your HTML body:
<ul class="w-96 text-sm font-medium text-gray-900 bg-white rounded-lg border border-gray-200 dark:bg-gray-700 dark:border-gray-600 dark:text-white mt-6">
<h3 class="text-xl font-bold ml-3 text-gray-300 uppercase py-2">Guilds:</h3>
<?php echo $guildMarkup;?>
</ul>
Finally, update your css file to get some of those new tailwind classes: