How to get a Minecraft Server's player count, MOTD, and status using Javascript
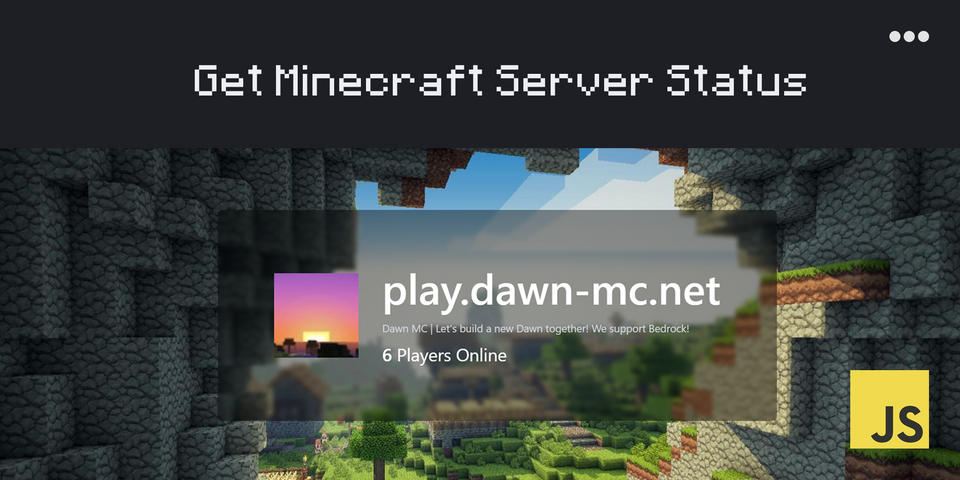
Getting the status of a Minecraft server is very easy using the free MCApi.us API. All it takes is hitting the endpoint with a basic fetch statement, and then parsing the data that is returned and displaying it on your html page.
Video Tutorial
Feel free to follow along with the video tutorial. I will be using this as a guide to reference code instead of writing it fully in the video.
Getting Started
In the starter code in the video, I use a HTML + CSS page that I made off camera. I wanted to keep the tutorial short in order to show how easy it us to plug this stuff in to your existing application. Please feel free to leave feedback if you are interested in how to create this screen. It was built using tailwind CSS.
Where to download started files:
The starter files can be found in the following github repository. Just navigate over to it,
Coding Tutorial
Starter HTML
<body>
<div>
<img src="https://cdn.pixabay.com/photo/2013/07/12/19/25/minecraft-154749_1280.png" id="server-icon"/>
<div>
<h1 id="server-ip">play.myserver.com</h1>
<span id="motd">A beautiful minecraft server</span>
<div><span id="player-count">290</span> Players Online</div>
</div>
</div>
</body>
Initializing the function
We are going to create a function that will accept the severIp, and serverPort as parameters. We will pass these to the API that will return back the information about the server.
function initServerData(serverIp,serverPort){
// our function will take 2 parameters
console.log(serverIp);
console.log(serverPort);
}
initServerData("play.dawn-mc.net","25565");
Utilizing the JavaScript fetch() API
The fetch() API lets us hit an external endpoint. We are expecting a json response, so we will automatically parse our data.
We are going to be using the MCApi.us free api. It returns a json object on the status of any server you decide to hit.
function initServerData(serverIp,serverPort){
fetch('https://mcapi.us/server/status?ip='+serverIp+'&port='+serverPort)
.then(response => response.json())
.then(data => console.log(data));
}
The official documentation, and support can be found on https://mcapi.us/#usage
Handling the data that we received
Let's write a function that will take in the data we receive, and output it throughout our HTML.
Although the object sends a lot more data, we will be expecting: an MOTD message, a player count, and a favicon.
This is a sample return json body:
{
"status": "success",
"online": true,
"motd": "Dawn MC | Let's build a new ...",
"motd_json": {
"extra": [
{
"color": "gold",
"text": "D"
},
{
"color": "gold",
"text": "a"
},
...
],
"text": ""
},
"favicon": "data:image/png;base64,iVBORw0KGgoAAA...",
"error": null,
"players": {
"max": 100,
"now": 9,
"sample": [
{
"name": "EchoLynne",
"id": "f1b98f4b-8c66-4d77-a53b-f8784c0607cf"
},
...
]
},
"server": {
"name": "Paper 1.18.2",
"protocol": 758
},
"last_updated": "1652491121",
"duration": "24093588"
}
As you can see, our relevant information is stored in returnData.motd, returnData.favicon, and returnData.players.now.
The cool thing is that you can get a lot more information out of this API. Such players.sample[] will return the actual individual players on the server, and the MOTD characters are literally colored in the motd_json.
Setting the HTML
function handleServerStatus(data){
const motd = document.getElementById("motd");
motd.innerHTML = data.motd;
const playerCounter = document.getElementById("player-count");
playerCounter.innerHTML = data.players.now;
const logo = document.getElementById("server-icon");
logo.src = data.favicon;
}
Now that we have all of our html elements selected (be sure to have the corresponding id="motd", etc. in your HTML, we can set their innerHTML to our data.
Error Handling
function handleServerStatus(data){
if(data.status=='error'){
console.log(data.error);
return false;
}
const motd = document.getElementById("motd");
motd.innerHTML = data.motd;
const playerCounter = document.getElementById("player-count");
playerCounter.innerHTML = data.players.now;
const logo = document.getElementById("server-icon");
logo.src = data.favicon;
}
Complete Script
<script>
function initServerData(serverIp,serverPort){
const serverIpElement = document.getElementById('server-ip');
serverIpElement.innerHTML = serverIp;
fetch('https://mcapi.us/server/status?ip='+serverIp+'&port='+serverPort)
.then(response => response.json())
.then(data => handleServerStatus(data));
function handleServerStatus(data){
if(data.status=='error'){
console.log(data.error);
return false;
}
const motd = document.getElementById("motd");
motd.innerHTML = data.motd;
const playerCounter = document.getElementById("player-count");
playerCounter.innerHTML = data.players.now;
const logo = document.getElementById("server-icon");
logo.src = data.favicon;
}
}
initServerData("play.dawn-mc.net","25565");
</script>