How to get a Discord User's Server Roles using PHP and Redirect With Simple Permissions
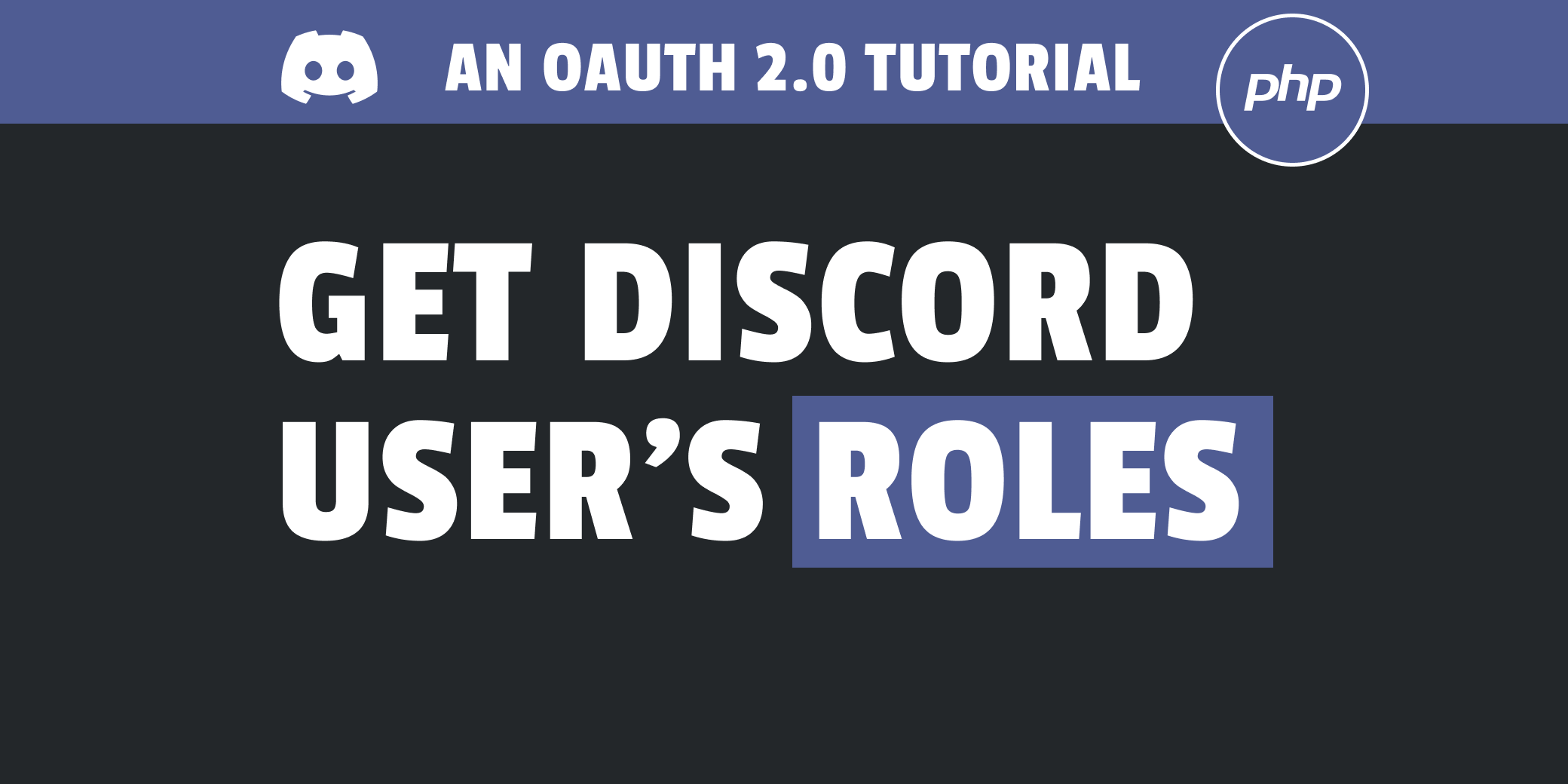
Here is the scenario. You want to log a user into your website, and then see if they have the required role (from your discord server) to view something on your site. In my example, I will redirect admins to a special page, moderators to their page, and the rest of users to a regular, login protected page.
Video Tutorial:
Source Code:
Check out the GitHub if you want to start with a plug-n-play template:
Getting Started:
Start with my Login with Discord (PHP) tutorial on YouTube. It's about 20 minutes, but will get you up to speed on how to set up your discord developer account, and make some API calls with PHP!
1) Update your OAuth URL
If you followed the tutorial, your redirect URL probably looks like this:
https://discord.com/api/oauth2/authorize?client_id=11349347517325240&redirect_uri=http%3A%2F%2Flocalhost%3A5000%2Fsrc%2Fprocess-oauth.php&response_type=code&scope=identify
Because we only picked the "scope" permission.
To get the user's roles, we need the guilds.members.read permission as well.
To add that into your URL, head on over to the Discord Developer Dashboard, and go to your application, and check the additional scope as seen here:
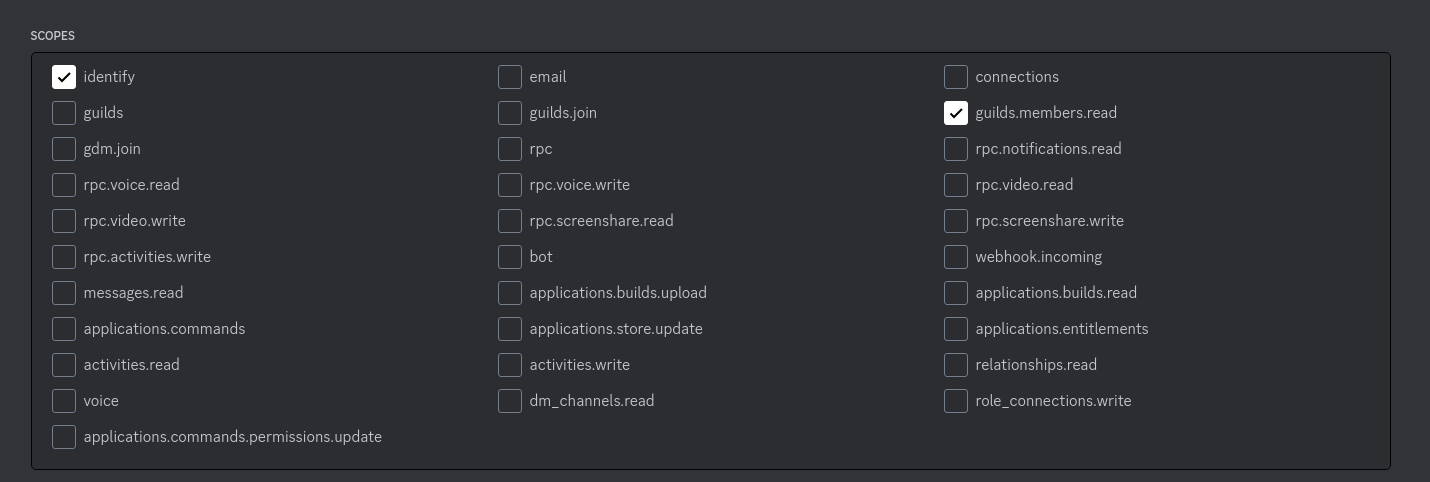
Now, copy the OAuth URL and paste it into your init-oauth.php file.
<?php
$discord_url = "https://discord.com/api/oauth2/authorize?client_id=1134934751732572240&redirect_uri=http%3A%2F%2Flocalhost%3A5000%2Fsrc%2Fprocess-oauth.php&response_type=code&scope=identify%20guilds%20guilds.members.read";
header("Location: $discord_url");
exit();
?>
2) Update your API Page (process-oauth.php)
Here is where we can now use our new permission to get information about the user's roles.
Get your server's guild id
Before we can do anything to find out the role of a user on a discord server, we needs its guild id.
Right click your discord server icon on discord, and select the bottom option "Copy Server ID"
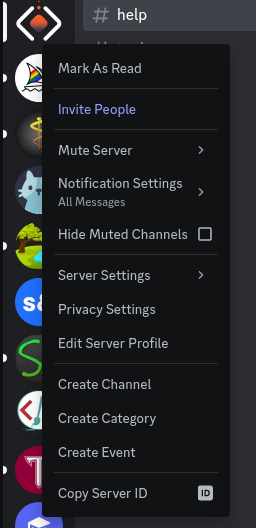
For example, mine is 98672642795098937
Get the user's role by passing the guild id
In process-oauth.php, let's make a function that takes in the access-token discord gave us, and search "our" roles on a discord server by passing it's guild id
function getGuildObject($access_token, $guild_id){
//requires the following scope: guilds.members.read
$discord_api_url = "https://discordapp.com/api";
$header = array("Authorization: Bearer $access_token","Content-Type: application/x-www-form-urlencoded");
$ch = curl_init();
//set the url, number of POST vars, POST data
curl_setopt($ch, CURLOPT_HTTPHEADER,$header);
curl_setopt($ch,CURLOPT_URL, $discord_api_url.'/users/@me/guilds/'.$guild_id.'/member');
curl_setopt($ch,CURLOPT_POST, false);
//curl_setopt($ch,CURLOPT_POSTFIELDS, $fields_string);
curl_setopt($ch,CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0);
$result = curl_exec($ch);
$result = json_decode($result,true);
return $result;
}
The guild object will return something like this...
Array
(
[avatar] =>
[communication_disabled_until] =>
[flags] => 0
[joined_at] => 2022-06-15T20:18:32.991000+00:00
[nick] =>
[pending] =>
[premium_since] =>
[roles] => Array
(
[0] => 1134940682650402968
)
[user] => Array
(
[id] => 118583685640355840
[username] => Exit
[avatar] => f2e98ae1156588254d4a6e3d9197fc8e
[discriminator] => 9063
[public_flags] => 0
[flags] => 0
[banner] =>
[accent_color] =>
[global_name] => Exit
[avatar_decoration] =>
[banner_color] =>
)
[mute] =>
[deaf] =>
[bio] =>
[banner] =>
)
Oh look! We have a roles array.
Let's access it, and test if it has a role that we care about.
$guild_roles = $guildObject['roles'];
// see if roles has the correct role_id within the array
$role = 'user';
if(in_array('1134940795481370646', $guild_roles)){
$role = 'admin';
}else if(in_array('1134940682650402968', $guild_roles)){
$role = 'moderator';
}
How do I know what 1134940795481370646 is?
Go to Server Settings > Roles >> Copy Role Id
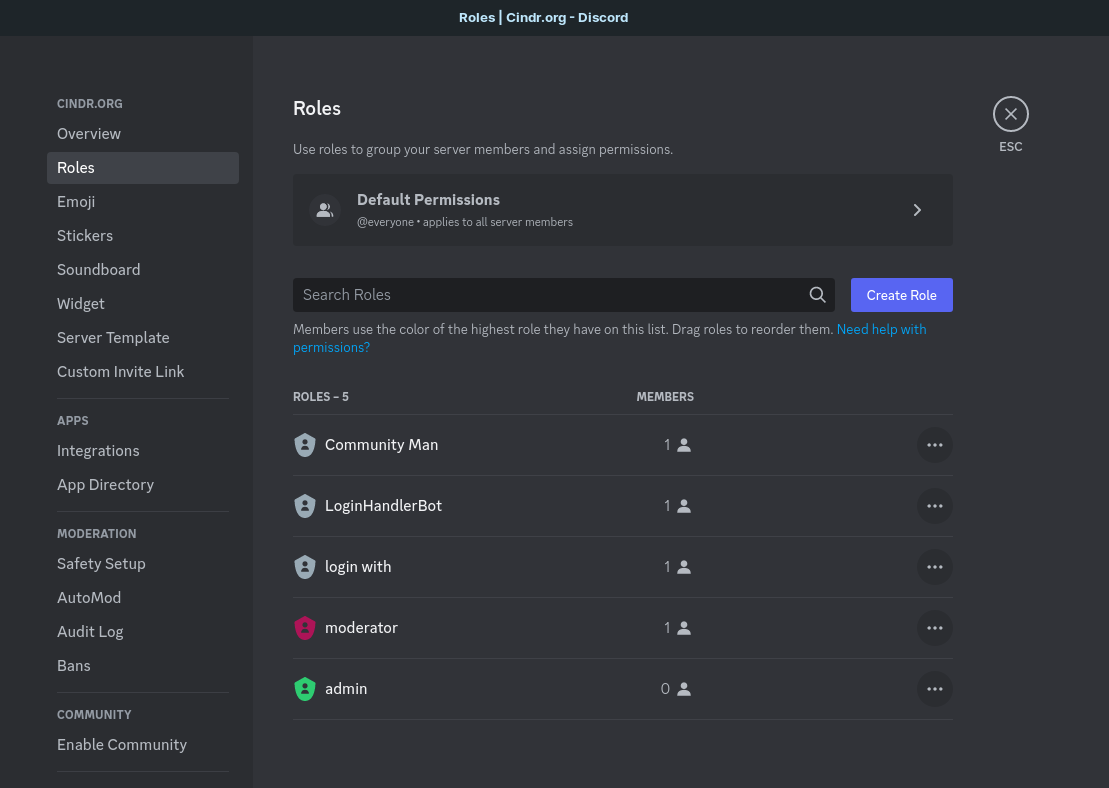
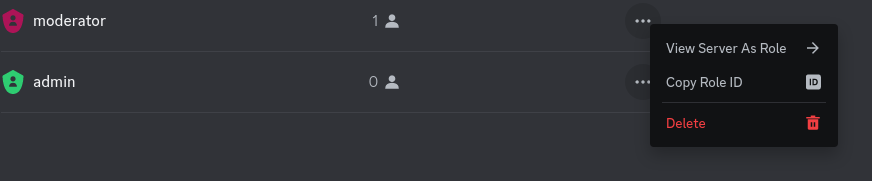
Ok, now let's update the user's session, so we can keep track of their role. Also, we can redirect them to their special, protected page!
$_SESSION['logged_in'] = true;
$_SESSION['userData'] = [
'name'=>$result['username'],
'discord_id'=>$result['id'],
'avatar'=>$result['avatar'],
'role'=>$role,
];
if($role=='admin'){
header("location: admin-lounge.php");
}else if($role=='moderator'){
header("location: moderator-lounge.php");
}else{
header("location: dashboard.php");
}
exit();
Here's an example of the admin-lounge.php page
<?php
session_start();
if($_SESSION['userData']['role']!='admin'){
header("location: index.php?error=not_admin");
exit();
}
?>
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="../dist/output.css" rel="stylesheet">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.1.1/css/all.min.css" integrity="sha512-KfkfwYDsLkIlwQp6LFnl8zNdLGxu9YAA1QvwINks4PhcElQSvqcyVLLD9aMhXd13uQjoXtEKNosOWaZqXgel0g==" crossorigin="anonymous" referrerpolicy="no-referrer" />
</head>
<body>
<div class='flex items-center justify-center h-screen font-bold'><?php echo 'Welcome, Admin'; ?></div>
</body>
</html>