How to Create a Simple Discord Bot using Python
This will be a short guide on creating a simple Discord bot using Python.
Initial Setup
We will need to install a few things and setup our workspace before we get started.
Install Python
The first ting we will need is Python, you can download the latest version from python.org or using your package manager on Linux.
Make sure to select Add Python to PATH when installing on Windows.
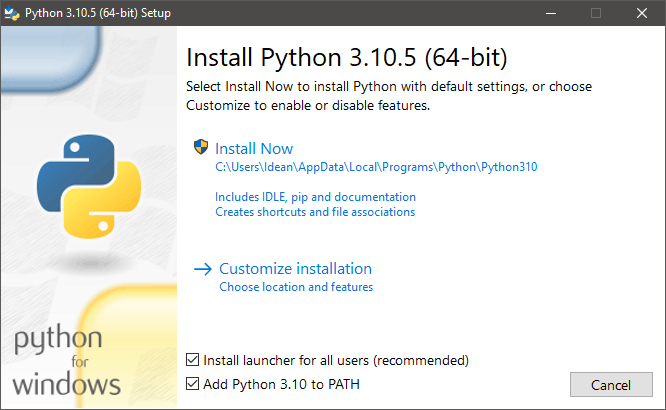
Setup Workspace
Install Discord.py
After installing python we can use pip to install Discord.py. Use one of the following commands depending on your OS and voice support.
Windows
py -3 -m pip install -U discord.py
py -3 -m pip install -U discord.py[voice]
Linux
python3 -m pip install -U discord.py
python3 -m pip install -U discord.py[voice]
On Linux you will need these dependencies for voice. You can use this command for Debian based systems. You can usually just install these with your package manager.
apt install libffi-dev libnacl-dev python3-dev
Create Bot Application
Next you will create a new application and bot account on the Discord Application Development Page.
Create a new application then in the application menu click Bot and then Add a new Bot account.
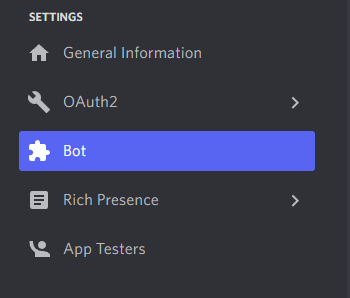
Once the bot account is created you will need to select the intents your Bot will need, you can change these later. But for your bot to respond to messages you should enable the Message Content Intent.
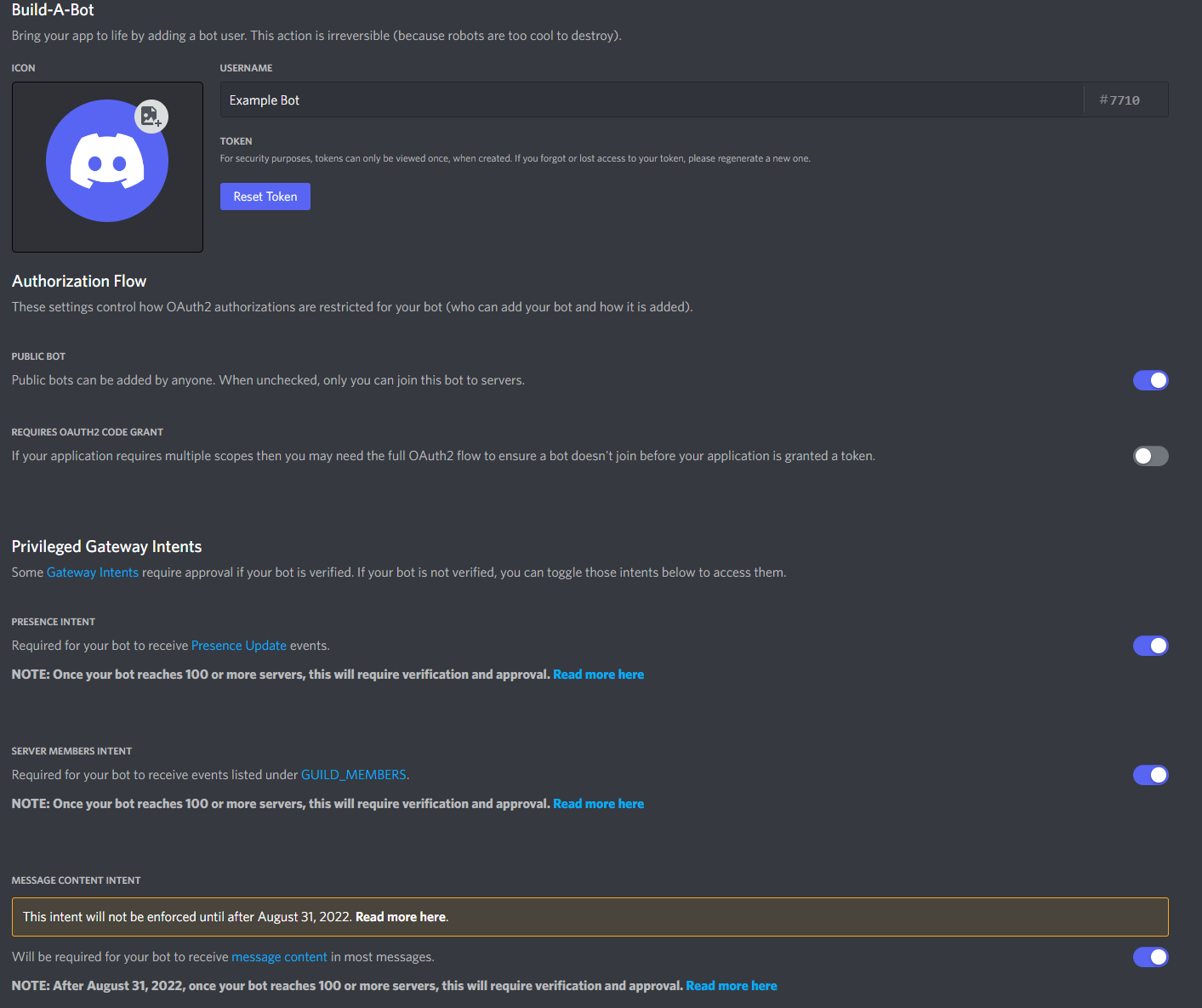
Finally copy the bot token. You will need this to run your bot.
Add Bot to Server
In the application settings menu click on OAuth2 then URL Generator.
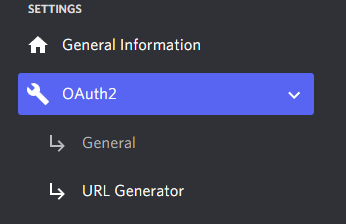
Under Scopes select Bot and copy the URL into your browser. You should get a popup allowing you to add your bot to your server.
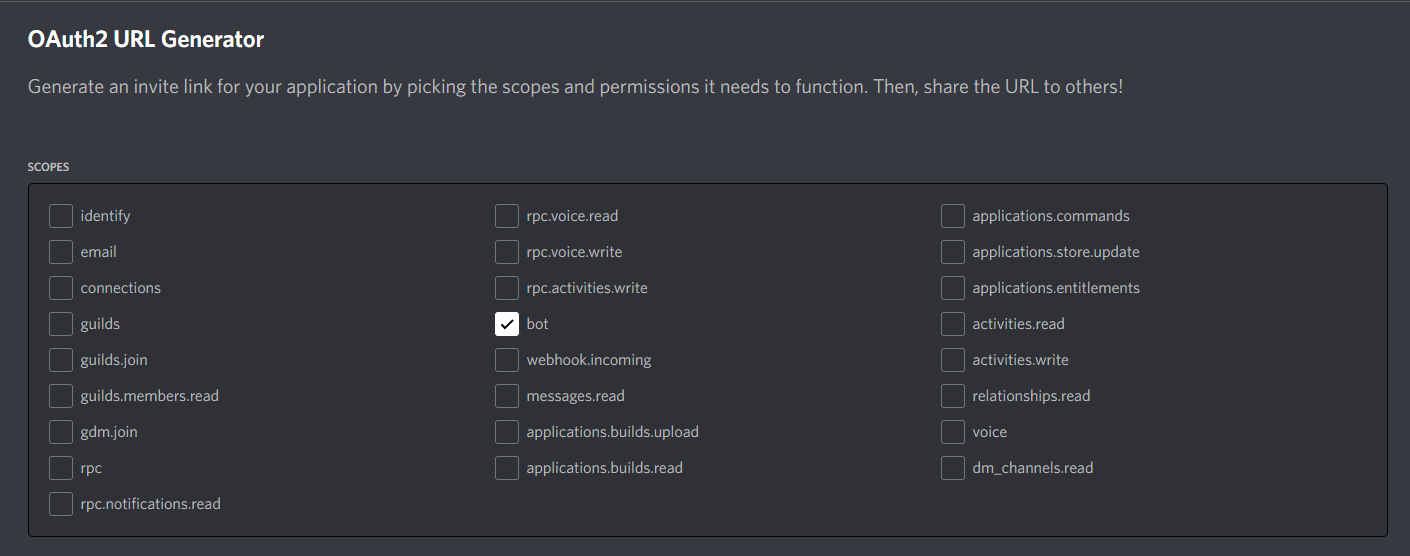
Create a Simple Bot
Basic Template
This is a starter template from the Discord.py Docs. Fill in your bot token and then run the file to start your bot.
import discord
class MyClient(discord.Client):
async def on_ready(self):
print(f'Logged on as {self.user}!')
async def on_message(self, message):
print(f'Message from {message.author}: {message.content}')
intents = discord.Intents.default()
intents.message_content = True
client = MyClient(intents=intents)
client.run('TOKEN HERE')
python bot.py
You should have your bot online now.