Create a simple sound output switch via Terminal in Linux & Bind it to a Key
Since I've switched from Windows to Linux, I've tried to make sure that all the tools I had on my Windows OS were available on Linux. I had a program called SoundSwitch that let me bind a hotkey to toggle through audio devices.
It was a simple program, but Linux makes this easy to do in a few lines.
I will be using Zorin OS for this tutorial as well.
Terminal Commands
The first command you need is:
pactl list sinks short | awk -F '\t' '{print $1,$2,$5}'
This will list your audio sources and extract out relavant information into line delimited list.
Mine outputs:
1 alsa_output.usb-Blue_Microphones_Yeti_Stereo_Microphone_REV8-00.iec958-stereo SUSPENDED
6 alsa_output.pci-0000_58_00.4.analog-stereo RUNNING
14 alsa_output.pci-0000_56_00.1.hdmi-stereo-extra4 SUSPENDED
Notice how there is an id, a reference name, and then its status. I realized that I want to switch between the Yeti output, and the analog-stero output.
The manual command for this is:
pacmd set-default-sink NUMBER_ID_OF_SINK
So for setting it back to my Yeti mic, I would execute:
pacmd set-default-sink 1
With this in mind, I have written a simple script to list out my inputs, get the selected input, and then swtich between the microphone or the speaker. Note, the IDs are dynamic, so you need to make sure you have a way to read/execute on them, and not just hard code in id=1, 6, 14, etc..
Making a bash script to switch between sources
#!/bin/bash
list=$(pactl list sinks short | awk -F '\t' '{print $1,$2,$5}')
selected_index_string=$(echo "$list" | grep "RUNNING")
yeti_index_string=$(echo "$list" | grep "Yeti")
stero_index_string=$(echo "$list" | grep "analog-stereo")
# Print the index of the line that has "RUNNING" in it
if [ "${selected_index_string}" == "${yeti_index_string}" ]; then
pacmd set-default-sink ${stero_index_string:0:2} # speakers
else
pacmd set-default-sink ${yeti_index_string:0:2} # microphone
fi
Adding a Keybind to execute the Script
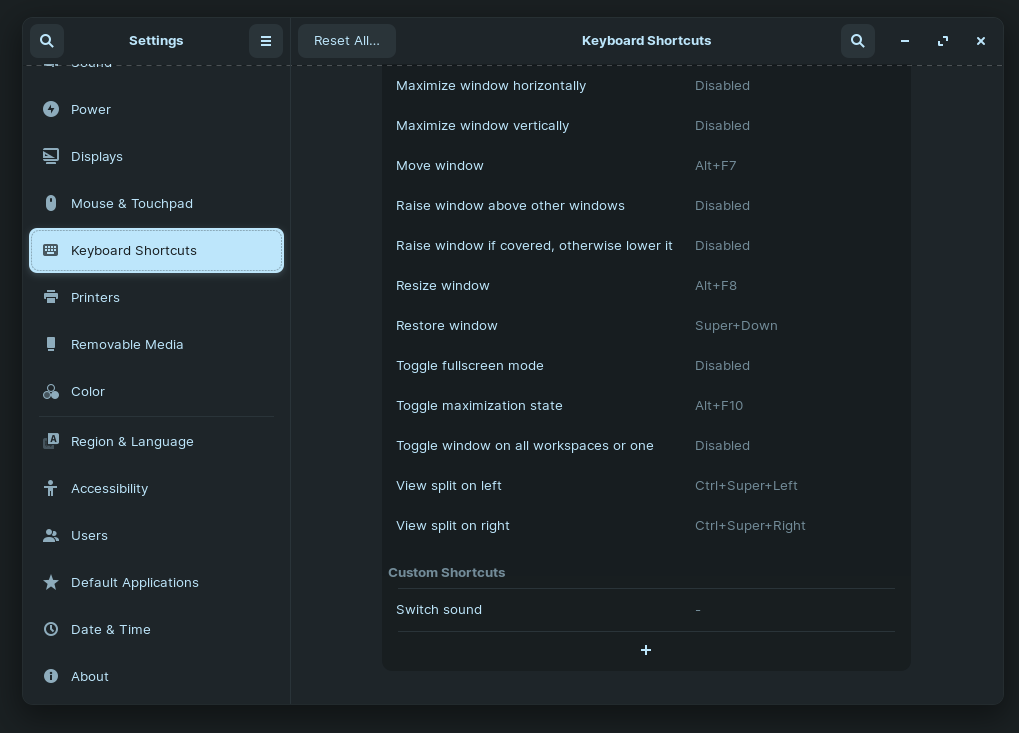
In ZorinOS go to settings, Keyboard Shortcuts, hit add new (the plus at the very bottom)
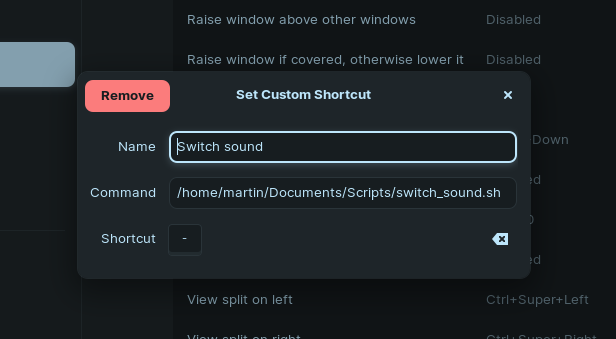
Name your shortcut, and give a path to the file
Make sure that you
chmod +x script_name.sh
To make it executable.
Thats it!